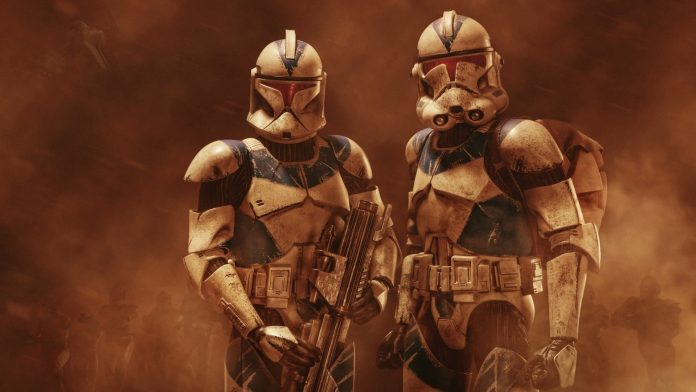
This is how you unload scripts and styles in WordPress:
Just add this code to your functions.php or to custom functions file:
// Dequeue a specific stylesheet
function dequeue_unused_styles() {
wp_dequeue_style( 'unused-stylesheet' );
wp_deregister_style( 'unused-stylesheet' );
}
add_action( 'wp_print_styles', 'dequeue_unused_styles', 100 );
// Dequeue a specific script
function dequeue_unused_scripts() {
wp_dequeue_script( 'unused-script' );
wp_deregister_script( 'unused-script' );
}
add_action( 'wp_print_scripts', 'dequeue_unused_scripts', 100 );
This code snippet uses the wp_dequeue_style()
and wp_deregister_style()
functions to dequeue and deregister a specific stylesheet, and the wp_dequeue_script()
and wp_deregister_script()
functions to dequeue and deregister a specific script. You need to replace “unused-stylesheet” and “unused-script” with the actual handle of the stylesheet and script you want to dequeue and deregister.
Here’s an example of how you can dequeue and deregister a specific stylesheet on only the “contact” page and a specific javascript file on only the “home” page:
add_action( 'wp_enqueue_scripts', 'my_dequeue_scripts' );
function my_dequeue_scripts() {
if ( is_page( 'contact' ) ) {
wp_dequeue_style( 'unwanted-stylesheet' );
wp_deregister_style( 'unwanted-stylesheet' );
}
if ( is_page( 'home' ) ) {
wp_dequeue_script( 'unwanted-javascript' );
wp_deregister_script( 'unwanted-javascript' );
}
}
In this example, I am using the is_page()
function to check if the current page is the “contact” page or “home” page, and if it is, then I am using the wp_dequeue_style()
and wp_deregister_style()
functions to remove the unwanted stylesheet and the wp_dequeue_script()
and wp_deregister_script()
functions to remove the unwanted javascript. You need to replace ‘unwanted-stylesheet’ and ‘unwanted-javascript’ with the handle of the stylesheet and javascript file you want to remove, respectively.
You can also use other conditional tags like is_home()
, is_front_page()
, is_single()
, is_singular()
etc. to target specific pages, posts or custom post types.
Please keep in mind that you should always test your changes on a development or staging site before applying them to a live site, as modifying the functions.php file can cause errors or conflicts if not done correctly.